Now, this is why i love the state of electronics at the moment – especially hobby grade ones. I can go from an idea on a piece of paper, to a working prototype, in… about 3 hours? Something like that. Anyway, in the time its taken since the last post, I have built and coded my Light Box controller, or at least prototyped it on a breadboard.
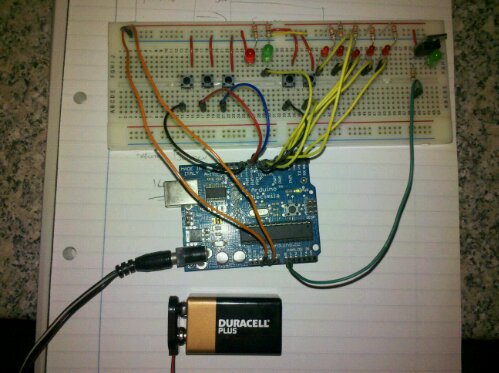
The breadboarding was actually the easy part – as you can see there are 5 buttons, 9 LED’s, a big Darlington Transistor, and an Arduino (and battery, but thats just for portable power). The buttons, from left to right are: Reset, Pause, Go, Up, and Down, and the LED’s are (again from left to right): Power, Go, 8 minutes, 4 minutes, 2 minutes, 1 minute, 30 seconds, and Array. The array LED is just as a place holder until I actually hook this up to the UV Array I have built (more on that in another post).
So when this is running, the timer counts down (in binary) in 30 second intervals. You can set it to the nearest 30 seconds using the up and down buttons – up to 15 minutes 30 seconds, and down to 30 seconds. The reset button sets it back to 8 minutes, although only when it is paused. The timer can be paused, and will turn off the UV Array when doing so, which would allow you to move or adjust something in the box. This will also allow for the simple addition of a switch to detect if the door is open, although in my current box that is pointless… the door never actually shuts.
The code for this is below (click the read more link to see it!)
//maximum time to run for - 480000 is 8 minutes. long maxTime = 480000; //The code is designed to work nicely for 8 minutes - changing this or the //step time divider will make the timing a bit more weird //Time for each LED step - there are enough LED's for atleast 31 but 16 is easier long stepTime = maxTime / 16; //current millis count at last check long startTime = 0; //remaining time in countdown long remainTime = maxTime; boolean running; //set all button pins int bReset = 12; int bPause = 11; int bGo = 10; int bUp = 9; int bDown = 8; //button debounce stuff for up and down int bUpState; int bUpStateLast = LOW; int bDownState; int bDownStateLast = LOW; int upDebounceTime = 0; int downDebounceTime = 0; int debounceDelay = 100; //set all LED pins int ledGo = 7; int led16 = 6; int led8 = 5; int led4 = 4; int led2 = 3; int led1 = 2; //set main array trigger pin int arrayUV = 14; //analog pin 0 void setup() { pinMode(bReset, INPUT); pinMode(bPause, INPUT); pinMode(bGo, INPUT); pinMode(bUp, INPUT); pinMode(bDown, INPUT); //turn on pulup resistors for buttons digitalWrite(bReset, HIGH); digitalWrite(bPause, HIGH); digitalWrite(bGo, HIGH); digitalWrite(bUp, HIGH); digitalWrite(bDown, HIGH); pinMode(ledGo, OUTPUT); pinMode(led16, OUTPUT); pinMode(led8, OUTPUT); pinMode(led4, OUTPUT); pinMode(led2, OUTPUT); pinMode(led1, OUTPUT); digitalWrite(ledGo, LOW); digitalWrite(led16, LOW); digitalWrite(led8, LOW); digitalWrite(led4, LOW); digitalWrite(led2, LOW); digitalWrite(led1, LOW); pinMode(arrayUV, OUTPUT); digitalWrite(arrayUV, LOW); remainTime = maxTime; } void loop() { if (!digitalRead(bGo) && !running) { startTime = millis() - (maxTime - remainTime); digitalWrite(ledGo, HIGH); digitalWrite(arrayUV, HIGH); running = true; } if (!digitalRead(bPause) && running) { remainTime = maxTime - (millis() - startTime); digitalWrite(arrayUV, LOW); digitalWrite(ledGo, LOW); running = false; } if (!digitalRead(bReset) && !running) { reset(); } if(running) { remainTime = maxTime - (millis() - startTime); } if (remainTime < 0) { reset(); } ledCounter(); if (!digitalRead(bUp) && !running && (remainTime = stepTime)) { remainTime = remainTime - stepTime; delay(200); } } void reset() { digitalWrite(arrayUV, LOW); digitalWrite(ledGo, LOW); remainTime = maxTime; running = false; } void ledCounter() { if ((remainTime / stepTime) % 2) { digitalWrite(led1, HIGH); } else { digitalWrite(led1, LOW); } if ((remainTime / (stepTime * 2)) % 2) { digitalWrite(led2, HIGH); } else { digitalWrite(led2, LOW); } if ((remainTime / (stepTime * 4)) % 2) { digitalWrite(led4, HIGH); } else { digitalWrite(led4, LOW); } if ((remainTime / (stepTime * 8)) % 2) { digitalWrite(led8, HIGH); } else { digitalWrite(led8, LOW); } if ((remainTime / maxTime) % 2) { digitalWrite(led16, HIGH); } else { digitalWrite(led16, LOW); } }